Some details
Code: A Summary
I’m not going to publish my whole Code here, but I will present a short explanation of how my App is structured.
Main Files:
- activity_main.xml – this displays a set of labelled Buttons, one for each Category of List.
- Main Activity – this contains the control-code for the Buttons, chooses which Activity to launch, and what message to send to it.
- Several Activities, for different types of List.
- Several Adaptor (or “Helper”) files, for processing the Lists, and binding them to the Recycler view.
- A “Class” file for each Category. These define an Object-Type, and show how each Object holds Data*.
- activity_(category).xml files, with data about how to display each List type
- (category)_list_row.xml files for showing how each Row of the List should display.
Why Multiple Category Files?
As this was my first real foray into Android Coding, I chose to split the Categories into two main types. Type The First were ones that could be express as “Names”. i.e. two pieces of Data. (Forename and Surname). This applied to Treasures (Type and Amount/Quality), Items (Alchemical, Mundane, Furniture all have Type and Material). Type The Second were ones that did not fit this structure, and each got it’s own set of Files/Classes/Activities/Helpers (Adaptors). e.g. Quests and Locations.
I could have piled up a single set of files with “IF <quest> then <do something different> else IF <Location> do <location code>”, but chose to separate them out for my ease of reading/maintaining.
What Objects Did You Use?
Each “category” was given it’s own Class (type of Object*).
<Deep breath …> Each time an item from a category was needed (each item on a list), I Instantiated a new Object of that Class:
To instantiate a new “Treasure” object:
Treasure thisTreasure = new Treasure();
This calls the Constructor of the Class, to create that instance of the Class (i.e. make that Object.)
e.g. Treasures are randomly chosen from “Coin”, “Art”, “Jewellery” and “Gem”. Each of these has a List of Types (Coins may be gold, silver or copper, Art may be a painting, a sculpture or a vase), and an Amount/Quality (Coins have a number, e.g. 20 Gold Crowns. A painting might be Elaborate or Exquisite, a Gem has a Cut).
Once instantiated, the Object has its set of Values, that can be called (e.g. by the Recycler Helper), to do something with (e.g. Display in a Recycler View).
Are you keeping up?
For the “Name”-type Objects (2 pieces of data each), they are all sent to the Name Adaptor to Display in the Name List (even if they are not names. It’s just what I called the 2-data section).
Quests and Locations got a little more complicated.
Each Quest Object holds several pieces of Data:
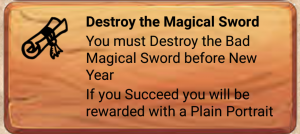
As you can see, each Quest is structured:
“You must <activity> the <descriptor> <item> before <time>. If you succeed, you will be rewarded with <reward>”
Each of these is called randomly from its String-Array. The Quest Adaptor must bind each part of this to the appropriate Recycler View.
As a complication, the Reward is called as a Treasure Object!
Locations were built in a similar manner.
Enough!
OK, that will do for today!
Let me know how much of that you understood, or if you would like some more explanations.
————-
*Java is an “Object Oriented Programming” (or “OOP”) language. Most things are “Objects”, that have “Methods” associated with them.