Life gets complicated.
Building Random Lists turned out to be a simple procedure. Buttons to choose which Category. Pick Lists (and grammar). Display in Recycler View.
I added a few extras, such as One Button to choose “Furniture”, with a sub-menu for Kitchen, Lounge, Bedroom etc. Quests included a Reward built from the Treasure Lists. Shops called from the Names Lists.
For the Pay Versions, I added some extra Features:
NPCs. Names became clickable, and built a random Person, with Traits pulled from other Lists. Personality, Valued Possession (Treasure), Mundane Items (new List), etc.
Add Data. This was a tricky one. I added the capability to add to the existing Lists. This data is stored in Text Files on the user’s phone, and added to the Array when the App draws up the Lists. I had quite a job figuring out where these files are stored, how to call them, and how to add them to the Array. In the end it worked though.
But we’re just calling Lists, and combining results.
Rome Wasn’t Built In a Day
And neither were my Apps!
But I did build a City-Builder. Not, unfortunately, a map-creator, although I am working on that. This is another Random Lists app that call up traits and features for a Fantasy City, such as Government Type, Local Features, Main Export, etc.

Again, each Feature was called from a List (still working with XML String-Arrays). Some used combined-lists (such as Renown). The Export facility was also included, and as can be seen from this picture, Exporting also saves a screen-shot.
A new feature for this App was to allow the User to “re-roll” a Trait. Clicking on one of the results will call up a new Trait.
The City is an “Object” (or “Class”) that holds information about each Trait, and when a Trait is re-rolled, the Class is updated with the new information. (Only one City is held at any time. Creating a new one over-writes the old one).
The City Names are created from two sections. One is a list of predetermined Names, and the other a prefix-suffix combo. Here we see “Pen” + “dale”. It could have produced “Pen”+”wood”, “Pen”+”ford”, etc. (As an aside, I am working on a Planet Creator, and those names can pull from an algorithm similar to the one used in Elite, that combines some syllables to form a real-sounding word. The Starport names draw from combos that include a “mixer” between the prefix and suffix. “Hadley’s Hope” might be “Hadley’s New Hope”, or Hadley’s Last Hope”).
Next …
I am reaching the limits of my imagination, and what can be done with Random Lists. While there are many more “skins” I could put on them (Dungeon, Modern City, Monsters, Furniture, you name it …), I need something to allow me to learn more about programming. One area I have been meaning to investigate is Databases. While I did some simple work on them for my RPG pages, I need to know how to fit them into Android!
An idea soon brews up: Random Loots! D&D has always included random Treasure Tables, so I could write something inspired by the latest version, saving all the raw data in in tables, and calling it as needed!
It turns out that the worst part of this is typing in all of the data! As with all of these apps, there is a lot of information that needs storing.
The main structure of the database was easy enough to build, using “DB Browser for SQLite“, although I did have to keep adding more columns as I realised what code I needed.
There are two types of Treasure: Individuals and Hoard. Individuals just carry Cash, which is easy to define the range (dependant upon Challenge Level) and roll some dice. Hoards can include Gems or Artworks, and the chance of Magical Items (OOooh!!). Roll on the Table to determine what sort of thing is in the Loot Pile, and then on each sub-table to find the details.

There are sub-tables for each Value of Gems and Artworks, and (as noted) multiple Magic Item tables. These are all easy to produce.
More difficult was deciding how much detail to display! Do the players want to know the full details of each gemstone, its exact value (for I introduced a randomiser for that!), what cut it has, etc, or just a Total Value for selling? I decided to include both! The App presents a Total, and clicks to present a list of details (using the previously-mentioned Recycler View).
New addition for Magic Item details was the Pop-Up window. This does not change what Activity you are in, but adds a new display over the top of it. Here I included Maker, Minor Power, and Quirks that the Item may have.
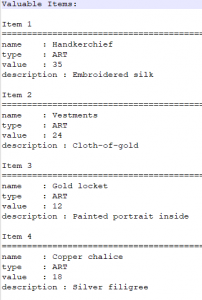
Finally the Export Code was added. Again, I used the Screenshot, but also looked at Formatting the Exported Text, so that it was easier to read. This mainly meant iterating the Treasure List and adding a few Line Breaks, with Section Titles.
With the addition of a few details to prettify the App (background Picture, Icons, nice buttons), it was ready to publish!
Finally
It may sound like a lot of this went smoothly, but I spent an inordinate amount of time struggling with sections of code, hunting typos, retyping functions and searching Google/StackOverflow for error-messages. Eventually, I have some workable Apps! You can download them here.
If you would like more detail about the actual Code I ended up with, or if you have ideas for new Apps you would like to see, ytou can contact me at:
admin@maddwarf.co.uk
Thank you.